Azure Key Vault - Update a Secret and its Properties Programmatically
When you use the Azure Key Vault SDK, you can create, read, update, and delete secrets. In this post, you will learn how to update a secret and its properties using the Azure Key Vault SDK.
I was working on the JosephGuadagno.NET Broadcasting application this weekend and wanted to create a feature that would automatically refresh the tokens that are used to authenticate with the various social media platforms. For reasons, these tokens were stored locally in the application settings. I know, bad choice, but when I started this project I really didn’t know Azure Key Vault, so I went with what I knew. I moved these tokens to Azure Key Vault, so now I needed to update the tokens in the Key Vault. However, when use Azure Key Vault SDK to update secrets, it creates an new version of the secret and doesn’t disable the previous secret. Since I am refreshing the token, the old token is no longer valid, so I needed to disable the old secret. At the same time, Azure Key Vaults secrets have an expiration date, so I wanted to update the expiration date of the secret as well. Let’s take a look at how to do this.
This post assumes you have an Azure Key Vault instance and you know how to setup a secret. If you don’t know how to set up a secret, you can follow the steps in the post Securing Azure Function Settings with Azure Key Vault.
The Process
There are a couple of steps to update a secret and its properties to achieve what I wanted to. The steps are:
- Establish a connection to the Azure Key Vault and obtain a
SecretClient
class. - Get the current secret from the vault using the
GetSecretAsync
method of theSecretClient
class. - Call the
UpdateSecretPropertiesAsync
method of theSecretClient
class to disable the secret. - Update / create a new version of the secret using the
SetSecretAsync
method of theSecretClient
class - Call the
UpdateSecretPropertiesAsync
method of theSecretClient
class using theKeyVaultSecret
returned from the step 4 to update the expiration date of the secret.
The Code
Here is the code to update a secret and its properties.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
public async Task UpdateSecretValueAndProperties(SecretClient client,
string secretName, string secretValue, DateTime expiresOn)
{
// Step 2: Get the current secret
var originalSecretResponse = await client.GetSecretAsync(secretName);
var originalSecret = originalSecretResponse.Value;
// Step 3: Disable the old secret
// Set the old secret to disabled
originalSecret.Properties.Enabled = false;
var updatePropertiesResponse =
await client.UpdateSecretPropertiesAsync(originalSecret.Properties);
// Step 4: Create a new version of the secret
// Update secret value (create a new version)
var newSecretVersionResponse = await client.SetSecretAsync(secretName, secretValue);
var newKeyVaultSecretVersion = newSecretVersionResponse.Value;
// Step 5: Update the expiration date
// Update the expiration date
newKeyVaultSecretVersion.Properties.ExpiresOn = expiresOn;
updatePropertiesResponse =
await client.UpdateSecretPropertiesAsync(newKeyVaultSecretVersion.Properties);
}
The error handling in this code was removed for brevity. You should add error handling to your code. You can find the full code in the jjgnet-broadcasting repository.
Wrap Up
I’m not sure why these steps are necessary to update a secret and its properties. I would think that the SetSecretAsync
method would update the secret and its properties with an overloaded method and/or better yet add an option to the SetSecretAsync
method to disable the previous version, if one exists. However, it doesn’t. I hope this post helps you if you need to update a secret and its properties in Azure Key Vault.
References
- Azure Key Vault
- Azure Key Vault SDK - SecretClient Class
- Azure Key Vault SDK - SecretClient.GetSecretAsync Method
- Azure Key Vault SDK - SecretClient.SetSecretAsync Method
- Azure Key Vault SDK - SecretProperties Class
- Azure Key Vault SDK - SecretClient.UpdateSecretPropertiesMethod
- Azure.Security.KeyVault.Secrets Documentation
Share on
Twitter Facebook LinkedIn RedditLike what you read?
Please consider sponsoring this blog.
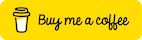